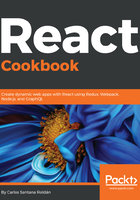
上QQ阅读APP看书,第一时间看更新
Basic animation – implementing componentWillUpdate
In this example, we are going to learn how to use componentWillUpdate:
- componentWillUpdate allows you to manipulate a component just before it receives new props or a new state. It is typically used for animations. Let's create a basic animation (fade in/fade out) to see how to use it:
import React, { Component } from 'react';
import './Animation.css';
class Animation extends Component {
constructor() {
super();
this.state = {
show: false
};
}
componentWillUpdate(newProps, newState) {
if (!newState.show) {
document.getElementById('fade').style = 'opacity: 1;';
} else {
document.getElementById('fade').style = 'opacity: 0;';
}
}
toggleCollapse = () => {
this.setState({
show: !this.state.show
});
}
render() {
return (
<div className="Animation">
<button onClick={this.toggleCollapse}>
{this.state.show ? 'Collapse' : 'Expand'}
</button>
<div
id="fade"
className={
this.state.show ? 'transition show' : 'transition'
}
>
This text will disappear
</div>
</div>
);
}
}
export default Animation;
File: src/components/Animation/Animation.js
- As you can see, we are validating the show state with newState and observe that it is true. Then we add opacity 0, and if it is false, we add opacity 1. An important thing I want to mention about componentWillUpdate is that you can't update the state (which means you are not able to use this.setState) in this method because it will cause another call to the same method, creating an infinite loop. Let's add some styles:
.Animation {
background: red;
}
.Animation .transition {
transition: all 3s ease 0s;
color: white;
padding-bottom: 10px;
}
.Animation .transition.show {
padding-bottom: 300px;
background: red;
}
File: src/components/Animation/Animation.css
- If you run the application, you will see this view:

- After you click on the button, you will see an animation with the text fading out, and the red div will be expanded, giving you this result:
