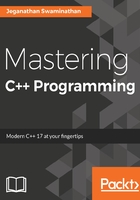
上QQ阅读APP看书,第一时间看更新
Vector
Vector is a quite useful sequence container, and it works exactly as an array, except that the vector can grow and shrink at runtime while an array is of a fixed size. However, the data structure used under the hood in an array and vector is a plain simple built-in C/C++ style array.
Let's look at the following example to understand vectors better:
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main () {
vector<int> v = { 1, 5, 2, 4, 3 };
cout << "\nSize of vector is " << v.size() << endl;
auto pos = v.begin();
cout << "\nPrint vector elements before sorting" << endl;
while ( pos != v.end() )
cout << *pos++ << "\t";
cout << endl;
sort( v.begin(), v.end() );
pos = v.begin();
cout << "\nPrint vector elements after sorting" << endl;
while ( pos != v.end() )
cout << *pos++ << "\t";
cout << endl;
return 0;
}
The preceding code can be compiled and the output can be viewed with the following commands:
g++ main.cpp -std=c++17
./a.out
The output of the program is as follows:
Size of vector is 5
Print vector elements before sorting
1 5 2 4 3
Print vector elements after sorting
1 2 3 4 5