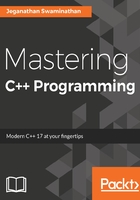
上QQ阅读APP看书,第一时间看更新
Multimap
A multimap works exactly as a map, except that a multimap container will allow multiple values to be stored with the same key.
Let's explore the multimap container with a simple example:
#include <iostream>
#include <map>
#include <vector>
#include <iterator>
#include <algorithm>
using namespace std;
int main() {
multimap< string, long > contacts = {
{ "Jegan", 2232342343 },
{ "Meena", 3243435343 },
{ "Nitesh", 6234324343 },
{ "Sriram", 8932443241 },
{ "Nitesh", 5534327346 }
};
auto pos = contacts.find ( "Nitesh" );
int count = contacts.count( "Nitesh" );
int index = 0;
while ( pos != contacts.end() ) {
cout << "\nMobile number of " << pos->first << " is " <<
pos->second << endl;
++index;
if ( index == count )
break;
}
return 0;
}
The program can be compiled and the output can be viewed with the following commands:
g++ main.cpp -std=c++17
./a.out
The output of the program is as follows:
Mobile number of Nitesh is 6234324343
Mobile number of Nitesh is 5534327346